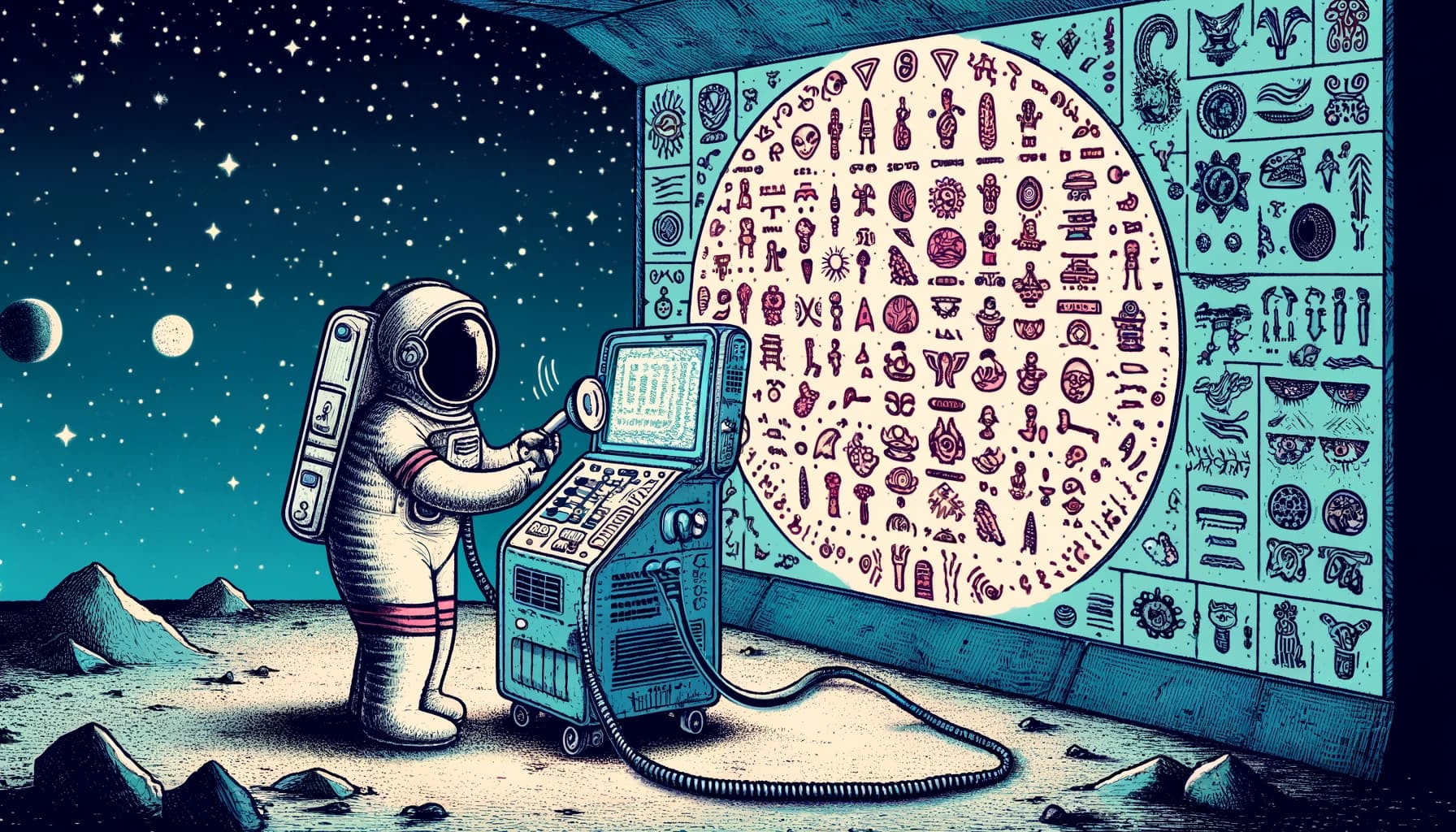
Typescript types that are friends not foe
TLDR
We improved Typescript types for results, added a short hand for querying on the ID of an entity, and added a useQueryOne hook to the React Client.
Typescript type improvements
Out of the box, Triplit now generates more human-readable types for your query results. Before when you inspected the type returned from a query you would get a type with an opaque name, with the latest update results will have simple types that are usually plain objects with simple primitive values.
Before 🤔
QueryFetchResult<string, ClientFetchResultEntity<...>>
After 🤩
{
id: string;
name: string;
age: number;
}
A shorthand for querying by ID
We've added a shorthand for querying by ID. Now you can use the id
method when building queries as a more concise way to query for an entity by its ID.
Before
triplit.query('todos').where('id', '=', 'the-todo-id');
After
triplit.query('todos').id('the-todo-id');
useQueryOne React hook
If you're building a React app with Triplit, you can now use the useQueryOne
hook when you only want a single entity returned from your query. This hook works the same as the useQuery
but it will only return a single entity instead of a multiple of entities. Going forward you should use this instead of the useEntity
hook.
Example
import { useQueryOne } from '@triplit/react';
import client from './triplit-client';
function AlbumPage({ albumId }: { albumId: string }) {
const query = client.query('albums').id(albumId).include('tracks');
const { result: albumWithTracks } = useQueryOne(client, query);
return <>...</>;
}